@RequestMapping("/getPhone")
public ResponseObject getPhone(String code) throws Exception {
log.info(code + ">>>>>>>>>>>>>>");
JSONObject token = JSON.parseObject(HttpClientUtils.doGet(WechatConstant.AUTH_ACCESS_TOKEN_URL));
if (token == null) {
log.info("获取token失败");
throw new BussinessException("获取token失败");
}
String accessToken = token.getString("access_token");
log.info(accessToken+">>>>>>>>>>>>>>>>>>>>>>>>>");
if (StringUtils.isEmpty(accessToken)) {
log.info("获取access_token失败");
throw new BussinessException("获取access_token失败");
}
Map jsonCode=new HashMap();
jsonCode.put("code", code);
System.out.println("jscode的值是"+jsonCode);
String resPhone = HttpUtil.post("https://api.weixin.qq.com/wxa/business/getuserphonenumber?access_token=" + accessToken, JSON.toJSONString(jsonCode));
log.info(resPhone);
if (StringUtils.isEmpty(resPhone) || !resPhone.contains("phone_info") || !resPhone.contains("phoneNumber")) {
log.info("测试用");
resPhone = HttpClientUtils.doPost("https://api.weixin.qq.com/wxa/business/getuserphonenumber?access_token=" + accessToken, JSON.toJSONString(jsonCode));
log.info(resPhone);
}
JSONObject resPhoneInfo = JSON.parseObject(resPhone);
JSONObject phoneInfo = resPhoneInfo.getJSONObject("phone_info");
System.out.println(resPhoneInfo);
System.out.println(phoneInfo);
String phoneNumber = phoneInfo.get("phoneNumber").toString();
return new ResponseObject(phoneNumber);
}
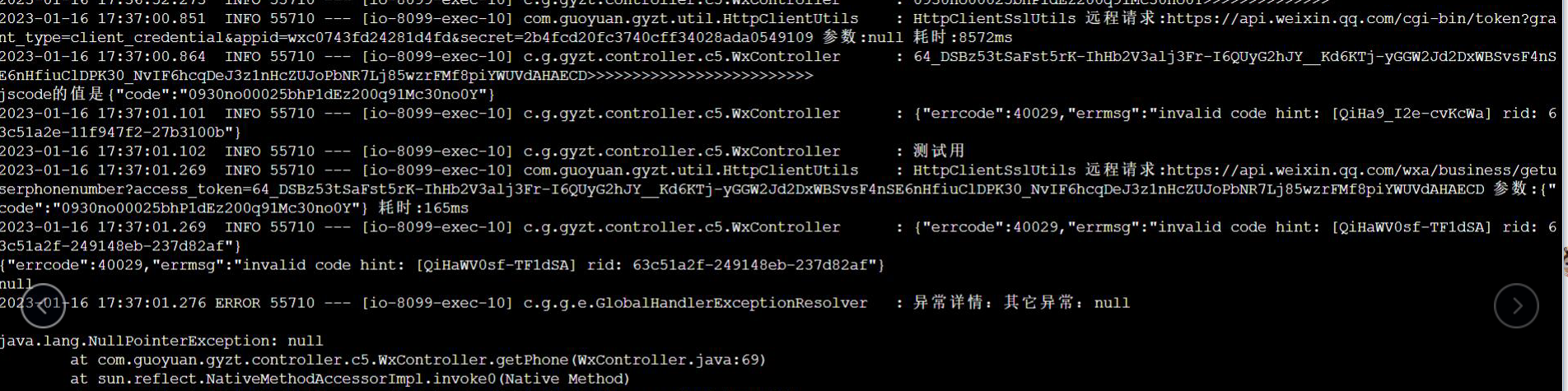
**
* @author 潘彬~
* @version2,0
* HttpUtil工具类
*/
@Slf4j
public class HttpClientUtils {
private static final String DEFAULT_CHAR_SET = "UTF-8";
private static final Integer DEFAULT_CONNECTION_TIME_OUT = 2000;
private static final Integer DEFAULT_SOCKET_TIME_OUT = 3000;
private static final Integer SOCKET_TIME_OUT_UPPER_LIMIT = 10000;
private static final Integer SOCKET_TIME_OUT_LOWER_LIMIT = 1000;
private static CloseableHttpClient getHttpClient() {
RequestConfig requestConfig = RequestConfig.custom().setSocketTimeout(DEFAULT_SOCKET_TIME_OUT)
.setConnectTimeout(DEFAULT_CONNECTION_TIME_OUT).build();
return HttpClients.custom().setDefaultRequestConfig(requestConfig)
.setRetryHandler(new DefaultHttpRequestRetryHandler()).build();
}
private static CloseableHttpClient getHttpClient(Integer socketTimeOut) {
RequestConfig requestConfig =
RequestConfig.custom().setSocketTimeout(socketTimeOut).setConnectTimeout(DEFAULT_CONNECTION_TIME_OUT)
.build();
return HttpClients.custom().setDefaultRequestConfig(requestConfig)
.setRetryHandler(new DefaultHttpRequestRetryHandler()).build();
}
public static String doPost(String url, String requestBody) throws Exception {
return doPost(url, requestBody, ContentType.APPLICATION_JSON);
}
public static String doPost(String url, String requestBody, Integer socketTimeOut) throws Exception {
return doPost(url, requestBody, ContentType.APPLICATION_JSON, null, socketTimeOut);
}
public static String doPost(String url, String requestBody, ContentType contentType) throws Exception {
return doPost(url, requestBody, contentType, null);
}
public static String doPost(String url, String requestBody, List<BasicHeader> headers) throws Exception {
return doPost(url, requestBody, ContentType.APPLICATION_JSON, headers);
}
public static String doPost(String url, String requestBody, ContentType contentType, List<BasicHeader> headers)
throws Exception {
return doPost(url, requestBody, contentType, headers, getHttpClient());
}
public static String doPost(String url, String requestBody, ContentType contentType, List<BasicHeader> headers,
Integer socketTimeOut) throws Exception {
if (socketTimeOut < SOCKET_TIME_OUT_LOWER_LIMIT || socketTimeOut > SOCKET_TIME_OUT_UPPER_LIMIT) {
log.error("socketTimeOut非法");
throw new Exception();
}
return doPost(url, requestBody, contentType, headers, getHttpClient(socketTimeOut));
}
public static String doPost(String url, String requestBody, ContentType contentType, List<BasicHeader> headers,
CloseableHttpClient client) throws Exception {
CloseableHttpResponse response = null;
long startTime = System.currentTimeMillis();
try {
HttpPost post = new HttpPost(url);
if (!CollectionUtils.isEmpty(headers)) {
for (BasicHeader header : headers) {
post.setHeader(header);
}
}
StringEntity entity =
new StringEntity(requestBody, ContentType.create(contentType.getMimeType(), DEFAULT_CHAR_SET));
post.setEntity(entity);
response = client.execute(post);
if (response.getStatusLine().getStatusCode() != HttpStatus.OK.value()) {
log.error("业务请求返回失败:{}", EntityUtils.toString(response.getEntity()));
throw new Exception();
}
String result = EntityUtils.toString(response.getEntity());
return result;
} finally {
releaseResourceAndLog(url, requestBody, response, startTime);
}
}
public static String doPostWithUrlEncoded(String url,
Map<String, String> param) throws Exception {
CloseableHttpClient httpClient = getHttpClient();
CloseableHttpResponse response = null;
long startTime = System.currentTimeMillis();
try {
HttpPost httpPost = new HttpPost(url);
if (param != null) {
List<org.apache.http.NameValuePair> paramList = new ArrayList<>();
for (String key : param.keySet()) {
paramList.add(new BasicNameValuePair(key, param.get(key)));
}
UrlEncodedFormEntity entity = new UrlEncodedFormEntity(paramList, DEFAULT_CHAR_SET);
httpPost.setEntity(entity);
}
response = httpClient.execute(httpPost);
if (response.getStatusLine().getStatusCode() != HttpStatus.OK.value()) {
log.error("业务请求返回失败:{}" , EntityUtils.toString(response.getEntity()));
throw new Exception();
}
String resultString = EntityUtils.toString(response.getEntity(), DEFAULT_CHAR_SET);
return resultString;
} finally {
releaseResourceAndLog(url, param == null ? null : param.toString(), response, startTime);
}
}
private static void releaseResourceAndLog(String url, String request, CloseableHttpResponse response, long startTime) {
if (null != response) {
try {
response.close();
recordInterfaceLog(startTime, url, request);
} catch (IOException e) {
log.error(e.getMessage());
}
}
}
public static String doGet(String url) throws Exception {
return doGet(url, ContentType.DEFAULT_TEXT);
}
public static String doGet(String url, ContentType contentType) throws Exception {
return doGet(url, contentType, null);
}
public static String doGet(String url, List<BasicHeader> headers) throws Exception {
return doGet(url, ContentType.DEFAULT_TEXT, headers);
}
public static String doGet(String url, ContentType contentType, List<BasicHeader> headers) throws Exception {
CloseableHttpResponse response = null;
long startTime = System.currentTimeMillis();
try {
CloseableHttpClient client = getHttpClient();
HttpGet httpGet = new HttpGet(url);
if (!CollectionUtils.isEmpty(headers)) {
for (BasicHeader header : headers) {
httpGet.setHeader(header);
}
}
if(contentType != null){
httpGet.setHeader("Content-Type", contentType.getMimeType());
}
response = client.execute(httpGet);
if (response.getStatusLine().getStatusCode() != HttpStatus.OK.value()) {
log.error("业务请求返回失败:{}", EntityUtils.toString(response.getEntity()));
throw new Exception();
}
String result = EntityUtils.toString(response.getEntity());
return result;
} finally {
releaseResourceAndLog(url, null, response, startTime);
}
}
private static void recordInterfaceLog(long startTime, String url, String request) {
long endTime = System.currentTimeMillis();
long timeCost = endTime - startTime;
MDC.put("totalTime", String.valueOf(timeCost));
MDC.put("url", url);
MDC.put("logType", "third-platform-service");
log.info("HttpClientSslUtils 远程请求:{} 参数:{} 耗时:{}ms", url, request, timeCost);
}
}
code不正确,这个code不是wx.login 方法获取,是getPhoneNumber方法获取的:https://developers.weixin.qq.com/miniprogram/dev/framework/open-ability/getPhoneNumber.html
不请求自己后端,小程序直接获取code,然后postman直接请求code2session
<button open-type="getPhoneNumber" bindgetphonenumber="getPhoneNumber"></button>