const app = getApp().globalData
const song = require('../../utils/song.js')
const Lyric = require('../../utils/lyric.js')
let watch;
Page({
data: {
playurl: '',
playIcon : 'icon-play',
cdCls: 'pause',
currentLyric: null,
currentLineNum : 0,
toLineNum: 0,
currentSong: null,
dotsArray: new Array(2),
currentDot: 0
},
onLoad: function (options) {
},
onReady: function () {
},
onShow: function () {
console.log('onshow')
this._init()
this._getBackPlayfileName().then((res)=>{
const current = res.res.currentPosition
this.data.currentLyric.stop()
this.data.currentLyric.seek(current * 1000)
}).catch(()=>{
})
},
_init: function () {
let songlist = (app.songlist.length && app.songlist) || wx.getStorageSync('songlist')
let currentSong = app.songlist[app.currentIndex] || (songlist && songlist[app.currentIndex])
let duration = currentSong && currentSong.duration
this.setData({
currentSong: currentSong,
duration: this._formaTime(duration),
songlist: songlist,
currentIndex: app.currentIndex
})
this._getPlayUrl(currentSong.mid)
this._getLyric(currentSong)
},
_getBackPlayfileName: function() {
return new Promise((resolve,reject) => {
wx.getBackgroundAudioPlayerState({
success: function (res) {
var dataUrl = res.dataUrl
let ret = dataUrl && dataUrl.split('?')[0].split('/')[3]
resolve({ret,res})
},
fail: function (e) {
let ret = false
reject(ret)
}
})
})
},
_getPlayUrl: function (songmidid) {
const _this = this
wx.request({
url: 'https://c.y.qq.com/base/fcgi-bin/fcg_music_express_mobile3.fcg?g_tk=5381&inCharset=utf-8&outCharset=utf-8¬ice=0&format=jsonp&hostUin=0&loginUin=0&platform=yqq&needNewCode=0&cid=205361747&uin=0&filename=C400${songmidid}.m4a&guid=3913883408&songmid=${songmidid}&callback=callback',
data: {
g_tk: 5381,
inCharset: 'utf-8',
outCharset: 'utf-8',
notice:0,
format:'jsonp',
hostUin:0,
loginUin:0,
platform:'yqq',
needNewCode:0,
cid:205361747,
uin:0,
filename:'C400${songmidid}.m4a',
guid:3913883408,
songmid:songmidid,
callback:'callback',
},
success: function(res) {
var res1 = res.data.replace("callback(","")
var res2 = JSON.parse(res1.substring(0,res1.length - 1))
const playurl = 'http://dl.stream.qqmusic.qq.com/${res2.data.items[0].filename}?vkey=${res2.data.items[0].vkey}&guid=3913883408&uin=0&fromtag=66'
_this._getBackPlayfileName().then((nowPlay) => {
if(!(res2.data.items[0].filename === nowPlay.ret)){
_this._createAudio(playUrl)
}
}).catch((err) => {
_this._createAudio(playUrl)
})
}
})
},
createAudio: function(playUrl) {
wx.playBackgroundAudio({
dataUrl: 'playUrl',
title:this.data.currentSong.name,
coverImgUrl: this.data.currentSong.image
})
wx.onBackgroundAudioPlay(() => {
this.setData({
playIcon:'icon-pause',
cdCls:'play'
})
})
wx.onBackgroundAudioPause(() => {
this.setData({
playIcon:'icon-play',
cdCls:'pause'
})
})
wx.onBackgroundAudioStop(() => {
app.currentIndex++
this._init()
})
const manage = wx.getBackgroundAudioManager()
manage.onTimeUpdate(() => {
this.setData({
currentTime: this._formaTime(manage.currentTime),
percent:manage.currentTime / this.data.currentSong.duration
})
})
},
_getLyric: function(currentSong) {
const _this = this
this._getBackPlayfileName().then((res) => {
const nowMid = res.ret.split('.')[0].replace('C400','')
if(!(nowMid === currentSong.mid)){
if(this.data.currentLyric) {
this.data.currentLyric.stop && this.data.currentLyric.stop()
}
_this._getLyricAction(currentSong)
}
}).catch(() => {
_this._getLyricAction(currentSong)
})
},
_getLyricAction: function (currentSong) {
console.log('获取歌词')
song._getLyric(currentSong.musicId).then((res) => {
if(res.data.showapi_res_body.ret_code == 0) {
const lyric = this._normalizeLyric(res.data.showapi_res_body.lyric)
const currentLyric = new Lyric(lyric,this.handleLyric)
this.setData({
currentLyric:currentLyric
})
this.data.currentLyric.play()
}else {
console.log('无歌词')
this.setData({
currentLyric: {
lines: [{txt: '暂无歌词'}]
},
currentText: ''
})
}
})
},
_normalizeLyric: function (lyric) {
return lyric.replace(/:/g, ':').replace(/ /g, '\n').replace(/./g, '.').replace(/ /g, '').replace(/-/g, '-').replace(/(/g, '(').replace(/)/g, ')')
},
handleLyric: function({lineNum,txt}) {
console.log(lineNum)
this.setData({
currentLineNum:lineNum,
currentText: txt
})
if (lineNum > 5) {
this.setData({
toLineNum:lineNum - 5
})
}
},
_formaTime: function (interval) {
interval = interval | 0
const minute = interval / 60 | 0
const second = this._pad(interval % 60)
return '${minute} : ${second}'
},
_pad(num, n = 2) {
let len = num.toString().length
while (len < n) {
num = '0' + num
len++
}
return num
},
prev: function() {
if ((app.currentIndex - 1) < 0) {
app.currentIndex = this.data.songslist.length - 1
this._init()
return
}
app.currentIndex && app.currentIndex--
this._init()
},
next: function() {
if((app.currentIndex + 1) == this.data.songslist.length) {
app.currentIndex = 0
this._init()
return
}
app.currentIndex++
this._init()
},
togglePlaying:function() {
wx.getBackgroundAudioPlayerState({
success: function(res) {
var status = res.status
if (status == 1) {
wx.pauseBackgroundAudio()
} else {
wx.playBackgroundAudio()
}
}
})
let timer = setInterval(() => {
if(this.data.currentLyric) {
this.data.currentLyric.togglePlay()
clearInterval(timer)
}
},20)
},
openList: function() {
if(!this.data.songslist.length) {
return
}
this.setData({
translateCls : 'uptranslate'
})
},
close: function() {
this.setData({
translateCls: 'downtranslate'
})
},
end: function() {
console.log(2)
},
playthis: function(e) {
const index = e.currentTarget.dataset.index
app.currentIndex = index
this._init()
this.close()
},
changeDot: function(e) {
this.setData({
currentDot: e.detail.current
})
},
onPullDownRefresh: function () {
},
})
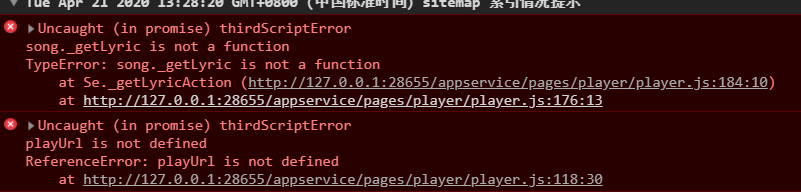
给出错误提示了都不看,哎
谢邀:
1、playurl和playUrl,U字大小写问题
应为:const playUrl
2、song._getLyric是不写错了,我看了,可能是this._getLyric?
如果不是。需要song.js源码定位问题
function Song({id,mid,singer,name,album,duration,image,musicId}) {
this.id = id
this.mid = mid
this.singer = singer
this.name = name
this.album = album
this.duration = duration
this.image = image
this.musicId = musicId
}
function createSong(musicData) {
return new Song({
id: musicData.songid,
mid: musicData.songmid,
singer: filterSinger(musicData.singer),
name: musicData.songname,
album: musicData.albumname,
duration: musicData.interval,
image:'https://y.gtimg.cn/music/photo_new/T002R300x300M000${musicData.albummid}.jpg?max_age=2592000',
musicId: musicData.songid
})
}
function filterSinger(singer) {
let ret = []
if (!singer) {
1.发一下 song.js的代码
2.注意大小写
如果觉得有帮助,请点个「有用」。千山万水总是情,别问「尾巴」行不行
这是song.js的代码
function Song({id,mid,singer,name,album,duration,image,musicId}) {
this.id = id
this.mid = mid
this.singer = singer
this.name = name
this.album = album
this.duration = duration
this.image = image
this.musicId = musicId
}
function createSong(musicData) {
return new Song({
id: musicData.songid,
mid: musicData.songmid,
singer: filterSinger(musicData.singer),
name: musicData.songname,
album: musicData.albumname,
duration: musicData.interval,
image:'https://y.gtimg.cn/music/photo_new/T002R300x300M000${musicData.albummid}.jpg?max_age=2592000',
musicId: musicData.songid
})
}
function filterSinger(singer) {
let ret = []
if (!singer) {
https://developers.weixin.qq.com/miniprogram/dev/devtools/minicode.html
如果觉得有帮助,请点个「有用」。千山万水总是情,别问「尾巴」行不行
如果觉得有帮助,请点个「有用」。千山万水总是情,别问「尾巴」行不行