<?php
header('Content-type:text/html; Charset=utf-8');
$mchid = 'xxxxx';
$appid = 'xxxxx';
$appKey = 'xxxxx';
$apiKey = 'xxxxx';
$outTradeNo = uniqid();
$payAmount = 0.01;
$orderName = '支付测试';
$notifyUrl = 'http://www.xxx.com/wx/notify.php';
$returnUrl = 'http://www.baidu.com';
$wapUrl = 'www.xxx.com';
$wapName = 'H5支付';
$wxPay = new WxpayService($mchid,$appid,$apiKey);
$wxPay->setTotalFee($payAmount);
$wxPay->setOutTradeNo($outTradeNo);
$wxPay->setOrderName($orderName);
$wxPay->setNotifyUrl($notifyUrl);
$wxPay->setReturnUrl($returnUrl);
$wxPay->setWapUrl($wapUrl);
$wxPay->setWapName($wapName);
$mwebUrl= $wxPay->createJsBizPackage($payAmount,$outTradeNo,$orderName,$notifyUrl);
echo "<h1><a href='{$mwebUrl}'>点击跳转至支付页面</a></h1>";
exit();
class WxpayService
{
protected $mchid;
protected $appid;
protected $apiKey;
protected $totalFee;
protected $outTradeNo;
protected $orderName;
protected $notifyUrl;
protected $returnUrl;
protected $wapUrl;
protected $wapName;
public function __construct($mchid, $appid, $key)
{
$this->mchid = $mchid;
$this->appid = $appid;
$this->apiKey = $key;
}
public function setTotalFee($totalFee)
{
$this->totalFee = $totalFee;
}
public function setOutTradeNo($outTradeNo)
{
$this->outTradeNo = $outTradeNo;
}
public function setOrderName($orderName)
{
$this->orderName = $orderName;
}
public function setWapUrl($wapUrl)
{
$this->wapUrl = $wapUrl;
}
public function setWapName($wapName)
{
$this->wapName = $wapName;
}
public function setNotifyUrl($notifyUrl)
{
$this->notifyUrl = $notifyUrl;
}
public function setReturnUrl($returnUrl)
{
$this->returnUrl = $returnUrl;
}
public function createJsBizPackage()
{
$config = array(
'mch_id' => $this->mchid,
'appid' => $this->appid,
'key' => $this->apiKey,
);
$scene_info = array(
'h5_info' =>array(
'type'=>'Wap',
'wap_url'=>$this->wapUrl,
'wap_name'=>$this->wapName,
)
);
$unified = array(
'appid' => $config['appid'],
'attach' => 'pay',
'body' => $this->orderName,
'mch_id' => $config['mch_id'],
'nonce_str' => self::createNonceStr(),
'notify_url' => $this->notifyUrl,
'out_trade_no' => $this->outTradeNo,
'spbill_create_ip' => $_SERVER['REMOTE_ADDR'],
'total_fee' => intval($this->totalFee * 100),
'trade_type' => 'MWEB',
'scene_info'=>json_encode($scene_info)
);
$unified['sign'] = self::getSign($unified, $config['key']);
$responseXml = self::curlPost('https://api.mch.weixin.qq.com/pay/unifiedorder', self::arrayToXml($unified));
$unifiedOrder = simplexml_load_string($responseXml, 'SimpleXMLElement', LIBXML_NOCDATA);
if ($unifiedOrder->return_code != 'SUCCESS') {
die($unifiedOrder->return_msg);
}
if($unifiedOrder->mweb_url){
return $unifiedOrder->mweb_url.'&redirect_url='.urlencode($this->returnUrl);
}
exit('error');
}
public static function curlPost($url = '', $postData = '', $options = array())
{
if (is_array($postData)) {
$postData = http_build_query($postData);
}
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $postData);
curl_setopt($ch, CURLOPT_TIMEOUT, 30);
if (!empty($options)) {
curl_setopt_array($ch, $options);
}
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false);
$data = curl_exec($ch);
curl_close($ch);
return $data;
}
public static function createNonceStr($length = 16)
{
$chars = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789';
$str = '';
for ($i = 0; $i < $length; $i++) {
$str .= substr($chars, mt_rand(0, strlen($chars) - 1), 1);
}
return $str;
}
public static function arrayToXml($arr)
{
$xml = "<xml>";
foreach ($arr as $key => $val) {
if (is_numeric($val)) {
$xml .= "<" . $key . ">" . $val . "</" . $key . ">";
} else
$xml .= "<" . $key . "><![CDATA[" . $val . "]]></" . $key . ">";
}
$xml .= "</xml>";
return $xml;
}
public static function getSign($params, $key)
{
ksort($params, SORT_STRING);
$unSignParaString = self::formatQueryParaMap($params, false);
$signStr = strtoupper(md5($unSignParaString . "&key=" . $key));
return $signStr;
}
protected static function formatQueryParaMap($paraMap, $urlEncode = false)
{
$buff = "";
ksort($paraMap);
foreach ($paraMap as $k => $v) {
if (null != $v) {
if ($urlEncode) {
$v = urlencode($v);
}
$buff .= $k . "=" . $v . "&";
}
}
$reqPar = '';
if (strlen($buff) > 0) {
$reqPar = substr($buff, 0, strlen($buff) - 1);
}
return $reqPar;
}
}
微信 支付宝 等支付方式都可以帮忙申请接入哦 我们属于微信 支付宝的服务商 也和很多持牌三方支付公司和银行合作的 需要的朋友欢迎咨询 遇到任何支付限额 关闭交易权限问题也可以咨询 赵18320304957
微信H5支付 只在手机百度极速版中 不好用 有没有解决办法 空白页
我有个问题请教:
2.商家参数格式有误,请联系商家解决(原因:当前调起H5支付的referer为空)
这里说的“当前调起H5支付的referer”是指PHP中通过curl发送的这个请求,还是只收到微信的支付URL在浏览器中发出的请求?如果是指后者,那应该如何设置referer呢?
appid是指服务号的appid吗,h5支付是否一定要认证服务号?
Node.js 版本可以参考 此篇文章
Java 版本可以参考 此篇文章
文章评论区有源码链接
如果对你有帮助请点击「有用」告知
simplexml_load_string前为什么不判断一下是不是空值,有没有请求成功呢
有个小问题,只是空值不参与签名,"null"字符串还是可以参与签名的。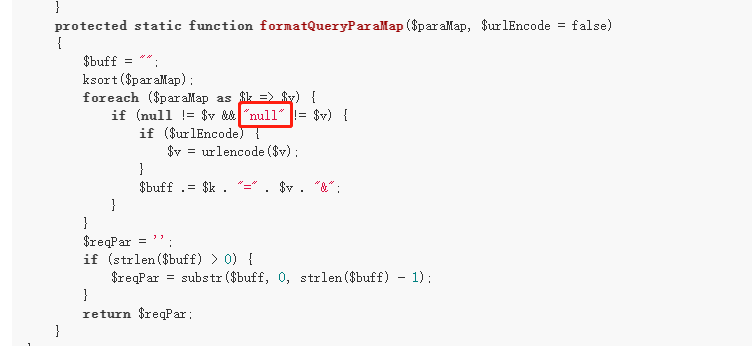