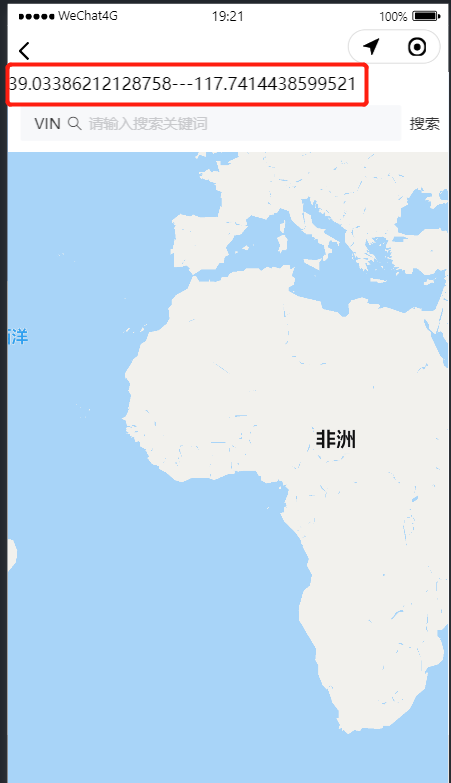
export default {
onLoad() { },
onShow: function () {
wx.setNavigationBarTitle({
title: "车辆定位",
});
},
data() {
return {
carNum: null,
points: [
],
maxSpeed: null,
scale: 3,
markers: [],
polyline: [{
points: [],
},],
latitude: 90,
longitude: 90,
centerPoint: {
latitude: 0,
longitude: 0
},
};
},
watch: {
points(e) {
let that = this;
if (that.points.length > 0) {
that.setDateByPoints(that.points);
}
},
},
async onShow() {
this.carNum = this.$root.$mp.query.carNum
await this.getPoints()
let that = this;
if (that.points.length > 0) {
that.setDateByPoints(that.points);
}
},
onUnload() {
this.carNum = ""
},
methods: {
onChange(val) {
this.carNum = val.mp.detail
},
query() {
this.getPoints()
},
getPoints() {
const json = {
url: "/api/customer/gps/getTrackList.do",
params: {
carNum: this.carNum,
beginTime: this.getBeforDate(),
endTime: this.getNowDate(),
},
success: (res) => {
if (res.data.code == 200) {
this.points = res.data.data
let arr = res.data.data
arr.forEach(element => {
element.latitude = element.lat
element.longitude = element.lng
});
this.latitude = arr[0].lat
this.longitude = arr[0].lng
}
},
fail: (res) => {
console.log(res);
},
};
this.ajax.get(json);
},
getNowDate() {
var timestamp = Date.parse(new Date());
var date = new Date(timestamp);
var Y = date.getFullYear();
var M = (date.getMonth() + 1 < 10 ? '0' + (date.getMonth() + 1) : date.getMonth() + 1);
var D = date.getDate() < 10 ? '0' + date.getDate() : date.getDate();
var h = date.getHours() <10 ? '0' +date.getHours() : date.getHours(),
m = date.getMinutes() < 10 ? '0'+date.getMinutes() : date.getMinutes(),
s = date.getSeconds() < 10 ? '0'+date.getSeconds() : date.getSeconds()
return Y + '-' + M + '-' +D + ' '+h+':'+m+':'+s;
},
getBeforDate() {
var timestamp = Date.parse(new Date()) - 3600 * 1000 * 24 * 15;
var date = new Date(timestamp);
var Y = date.getFullYear();
var M = (date.getMonth() + 1 < 10 ? '0' + (date.getMonth() + 1) : date.getMonth() + 1);
var D = date.getDate() < 10 ? '0' + date.getDate() : date.getDate();
var h = '00', m= '00', s = '00'
return Y + '-' + M + '-' + D+' ' +h+':'+m+':'+s;
},
computePointsSpeed(points) {
let lineColor = '#ffd500'
let list = []
if (!points || !points.length) {
return list
}
let lastArr = []
let lastSpeed = 0
for (let i = 0; i < points.length; i++) {
let gpsSpeed = points[i].gpsSpeed
if (!this.maxSpeed) {
this.maxSpeed = points[i]
} else {
if (points[i].gpsSpeed > this.maxSpeed.gpsSpeed) {
this.maxSpeed = points[i]
}
}
if (i === points.length - 1 || !gpsSpeed) {
continue
}
let nextPoint = points[i + 1]
let nextSpeed = points[i + 1].gpsSpeed
if (!nextSpeed) {
continue
}
lastSpeed = gpsSpeed
if (!lastArr.length) {
lastArr.push(points[i], nextPoint)
} else {
lastArr.push(nextPoint)
}
if (gpsSpeed <= 20) {
lineColor = '#ffd500'
if (nextSpeed > 20) {
list.push({
points: lastArr,
color: lineColor,
arrowLine: true,
width: 8,
})
lastArr = []
}
}
if (gpsSpeed > 20 && gpsSpeed <= 40) {
lineColor = '#ff8800'
if (nextSpeed <= 20 || nextSpeed > 40) {
list.push({
points: lastArr,
color: lineColor,
arrowLine: true,
width: 8,
})
lastArr = []
}
}
if (gpsSpeed > 40 && gpsSpeed <= 60) {
lineColor = '#ff5d00'
if (nextSpeed <= 40 || nextSpeed > 60) {
list.push({
points: lastArr,
color: lineColor,
arrowLine: true,
width: 8,
})
lastArr = []
}
}
if (gpsSpeed > 60 && gpsSpeed <= 80) {
lineColor = '#ff4d00'
if (nextSpeed <= 60 || nextSpeed > 80) {
list.push({
points: lastArr,
color: lineColor,
arrowLine: true,
width: 8,
})
lastArr = []
}
}
if (gpsSpeed > 80 && gpsSpeed <= 100) {
lineColor = '#ff3d00'
if (nextSpeed <= 80 || nextSpeed > 100) {
list.push({
points: lastArr,
color: lineColor,
arrowLine: true,
width: 8,
})
lastArr = []
}
}
if (gpsSpeed > 100 && gpsSpeed <= 120) {
lineColor = '#ff2d00'
if (nextSpeed <= 100 || nextSpeed > 120) {
list.push({
points: lastArr,
color: lineColor,
arrowLine: true,
width: 8,
})
lastArr = []
}
}
if (gpsSpeed > 120) {
lineColor = '#ff1d00'
if (nextSpeed <= 120) {
list.push({
points: lastArr,
color: lineColor,
arrowLine: true,
width: 8,
})
lastArr = []
}
}
}
this.centerPoint = points[Math.round(points.length / 2)]
console.log("centerPoint", this.centerPoint)
if (!list.length && lastArr.length) {
list.push({
points: lastArr,
color: lineColor,
arrowLine: true,
width: 8,
})
}
return list
},
setDateByPoints(points) {
let that = this;
let color = "#ffd500"
console.log(points, "---setDateByPoints--")
that.polyline = this.computePointsSpeed(points)
if (!that.polyline.length) {
that.polyline = [{
points: points,
color: color,
arrowLine: true,
width: 8,
}]
}
if (that.maxSpeed) {
that.maxSpeed.width = 24
that.maxSpeed.height = 24
that.maxSpeed.id = 2
that.maxSpeed.callout = {
color: '#5d5d5d',
fontSize: 14,
borderRadius: 6,
padding: 8,
bgColor: '#fff',
content: `极速 ${this.maxSpeed.gpsSpeed} km/h`
}
that.markers.push(this.maxSpeed)
}
let start = points[0]
let end = points[points.length - 1]
start.id = 1
start.width = 35
start.height = 35
end.id = 3
end.width = 35
end.height = 35
that.markers.push(start, end);
that.setCenterPoint(start, end)
},
setCenterPoint(start, end) {
this.longitude = (start.longitude + this.centerPoint.longitude + end.longitude) / 3
this.latitude = (start.latitude + this.centerPoint.latitude + end.latitude) / 3
let distance1 = this.getDistance(start.latitude, start.longitude, this.centerPoint.latitude, this.centerPoint.longitude)
let distance2 = this.getDistance(this.centerPoint.latitude, this.centerPoint.longitude, end.latitude, end.longitude)
const distance = Number(distance1) + Number(distance2)
console.log('计算两点之间的距离', distance1, distance2, distance)
if (distance < 200) {
this.scale = 17
}
if (distance >= 200 && distance < 1000) {
this.scale = 15
}
if (distance >= 1000 && distance < 5000) {
this.scale = 13
}
if (distance >= 5000 && distance < 10000) {
this.scale = 12
}
if (distance >= 10000 && distance < 15000) {
this.scale = 11
}
if (distance >= 15000 && distance < 50000) {
this.scale = 10
}
if (distance >= 50000 && distance < 200000) {
this.scale = 8
}
if (distance > 200000) {
this.scale = 5
}
},
getDistance: function (lat1, lng1, lat2, lng2) {
let rad1 = lat1 * Math.PI / 180.0;
let rad2 = lat2 * Math.PI / 180.0;
let a = rad1 - rad2;
let b = lng1 * Math.PI / 180.0 - lng2 * Math.PI / 180.0;
let r = 6378137;
return (r * 2 * Math.asin(Math.sqrt(Math.pow(Math.sin(a / 2), 2) + Math.cos(rad1) * Math.cos(rad2) * Math.pow(Math
.sin(b / 2), 2)))).toFixed(0)
},
},
};
请具体描述问题出现的流程,并提供能复现问题的简单代码片段(https://developers.weixin.qq.com/miniprogram/dev/devtools/minicode.html)。
@社区运营同学