UIToggle, UIToggleGroup components
Overview
UIToggle
is a radio button for simple state switching, and it needs to be used with TouchInputComponent
.
UIToggleGroup
is a UIToggle manager, used to adjust the state of other UIToggle when the state of any child UIToggle in the group changes.
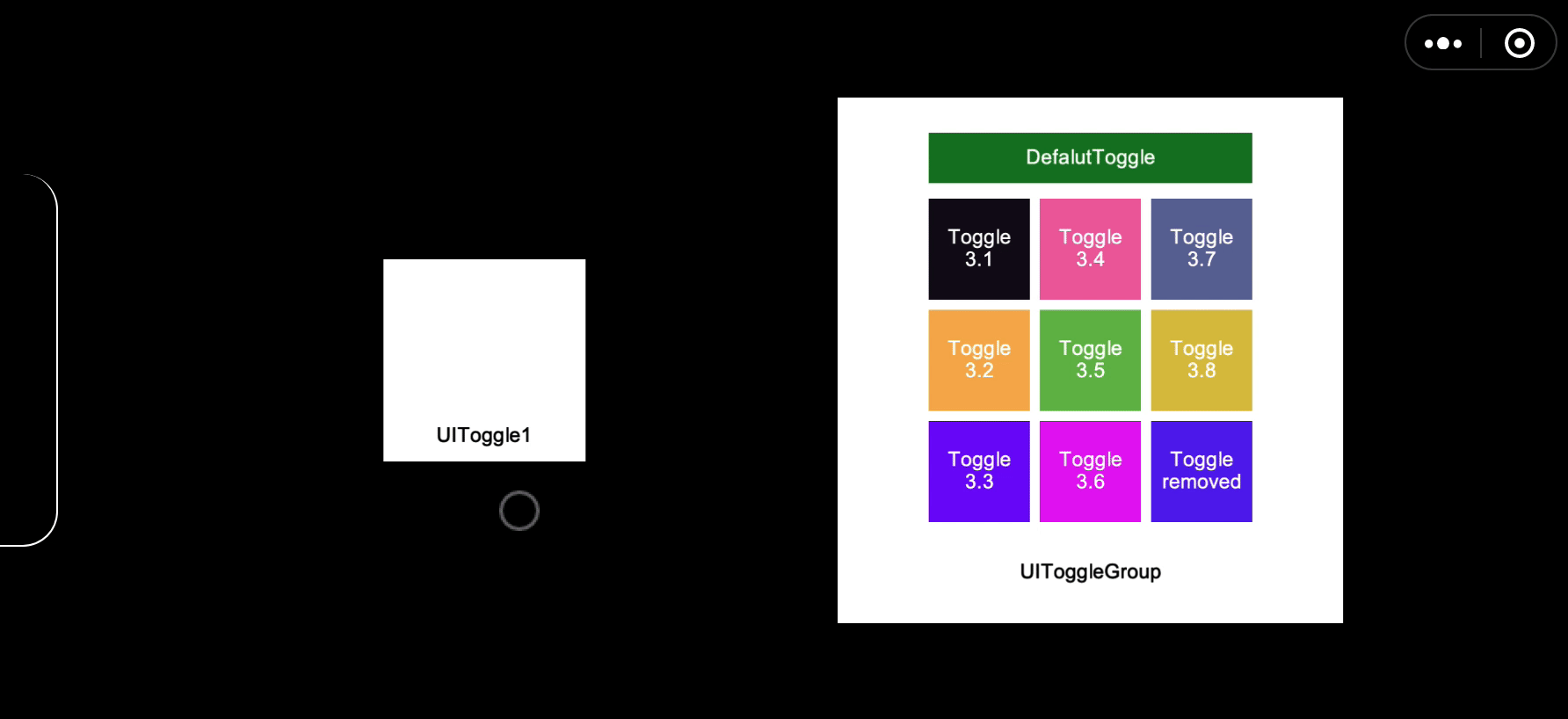
Static attribute description
ToggleState: UIToggle state enumeration value
UIToggle Property Description
Property name | Type | Default value | Description |
allowSwitchOff | boolean | true | Whether to allow deactivation. |
isChecked | boolean | false | Is it active? |
target | Renderable2D | undefined | Rendering components affected by UIToggle activation |
toggleGroup | UIToggleGroup | undefined | UIToggleGroup associated with UIToggle |
index | number | -1 | The index located in ToggleGroup, or -1 if there is no corresponding ToggleGroup |
UIToggle method description
Method | Parameters | Return Value | Function |
getState | | <ToggleState> | Return the current UIToggle state |
setState | <ToggleState> | Void | Set UIToggle state, which will affect isChecked |
UIToggleGroup Property Description
Property name | Type | Default value | Description |
allowSwitchOff | boolean | false | Whether the UIToggle under the UIToggleGroup is allowed to be deactivated. |
toggleList | Array<UIToggle> | [] | Read-only attribute, UIToggle array controlled by UIToggleGroup. |
deafaultActiveToggle | UIToggle | undefined | UIToggle in the UIToggleGroup that is activated by default. |
UIToggleGroup method description
Method | Parameters | Return Value | Function |
getActiveToggle | | <UIToggle / null> | Toggle that returns the current ToggleGroup activation state |
setIndex | <index: number> | <boolean> | According to index, activate Toggle in ToggleGroup and return whether activation is successful |
checkedChange | <toggle: UIToggle> | Void | Deactivate other toggles in the ToggleGroup except toggle |
addToggle | <toggle: UIToggle> | Void | Add UIToggle to the current UIToggleGroup |
removeToggle | <toggle: UIToggle> | Void | Delete UIToggle from current UIToggleGroup |
Notice
- If UIToggle is
no Target specified
, it will default to the first child node of the node where the Toggle is located that has a rendering component as the target of state switching, and the default state is display. - OnTouchEnter will set the isChecked of the current component to true.
- The UIToggle state change will trigger the
onStateChange(isChecked: Booleen, state: ToggleState)
of all components of the current node.